有了它,前端同学终于可以硬气的干活了
json-server 是一个 Node 模块,运行 Express 服务器,你可以指定一个 json 文件作为 api 的数据源。
json-server 可以直接把一个 json 文件托管成一个具备全RESTful 风格的 API,并
- 支持跨域
- jsonp
- 路由订制
- 数据快照保存
等功能的 web 服务器
安装
npm install -g json-server #一定要全局安装
新建一个 db.json 文件,并写入些数据
{
"posts": [{ "id": 1, "title": "json-server", "author": "typicode" }],
"comments": [{ "id": 1, "body": "some comment", "postId": 1 }],
"profile": { "name": "typicode" }
}
启动 JSON Server
json-server --watch db.json --port 8001 #监听8001端口
在浏览器里面输入 http://localhost:8001/posts
, 你会看到
{ "id": 1, "title": "json-server", "author": "typicode" }
项目中使用
默认已经全局安装了 json-server 插件.
在项目中新建 mock 文件夹,并且在mock文件夹中,新建 db.json 文件。
文件目录如下:
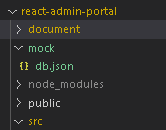
react-admin-portal\mock\db.json
{
"posts": [{ "id": 1, "title": "json-server", "author": "typicode" }],
"comments": [
{ "id": 1, "body": "some comment", "postId": 1 },
{ "id": 1, "body": "some comment", "postId": 1 },
{ "id": 1, "body": "some comment", "postId": 1 },
{ "id": 1, "body": "some comment", "postId": 1 },
{ "id": 1, "body": "some comment", "postId": 1 }
],
"profile": { "name": "typicode" }
}
在 mock 文件夹下,打开终端并执行如下命令
json-server --watch ./db.json -port 8001
终端显示如下图:(则是代表运行成功)
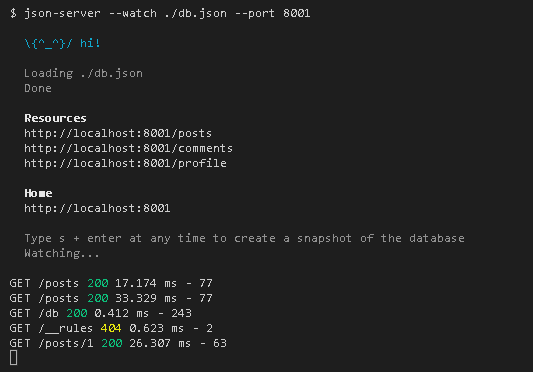
在浏览器“http://localhost:8001/”,则会出现下图中界面
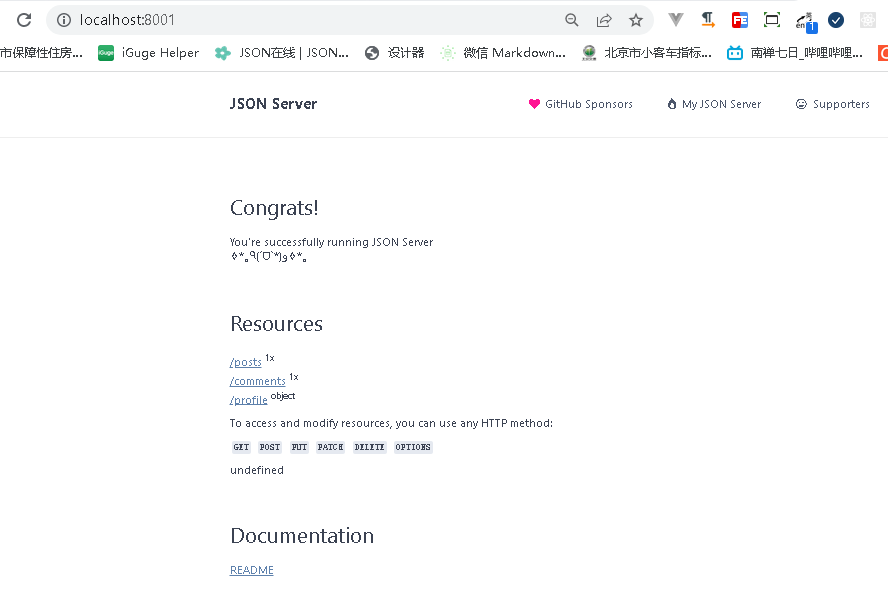
组件中使用
fetch 的基本方式
fetch(url, {
method: "GET", //'POST','PUT','DELETE'
headers: {
"Content-Type": "application/json", //'application/x-www-form-urlencoded'
Accept: "application/json",
},
body: JSON.stringfiy(body),
})
.then((res) => {
return res.json(); //返回一个Promise,解析成JSON,具体请看下面返回的数据
})
.then(function (res) {
console.log(res); //获取json数据
})
.catch(function (error) {
console.log(error); //请求错误时返回
});
获取数据
- 取全部数据 “http://localhost:8001/comments”
- 取部分数据 “http://localhost:8001/comments?id=1” 或者 “http://localhost:8001/comments/31”
// 获取数据
const loadData = () => {
fetch("http://localhost:8001/comments", {
method: "GET",
})
.then((res) => {
return res.json(); //返回一个Promise,解析成JSON,具体请看下面返回的数据
})
.then(function (res) {
console.log(res); //获取json数据
})
.catch(function (error) {
console.log(error); //请求错误时返回
});
};
结果展示
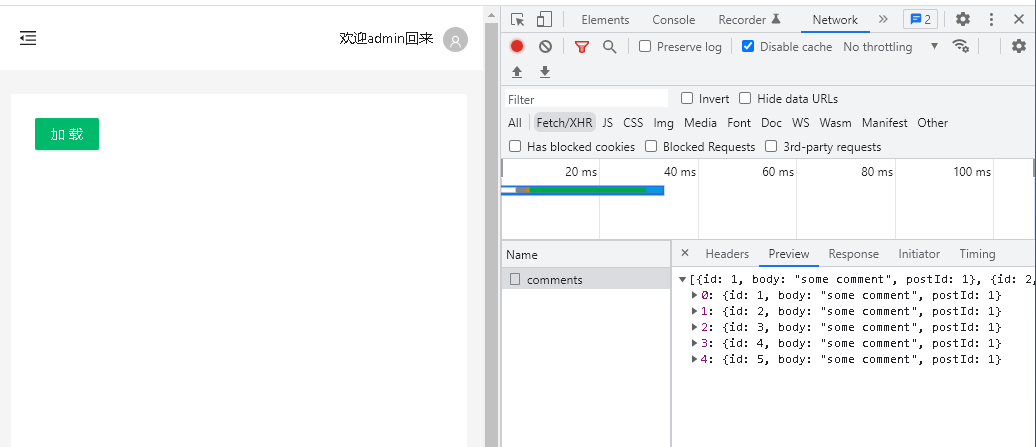
插入数据
const addData = () => {
fetch("http://localhost:8001/comments", {
method: "POST",
headers: {
"Content-Type": "application/json",
},
body: window.JSON.stringify({ id: 61, body: "some comment", postId: 1 }),
})
.then((res) => {
return res.json(); //返回一个Promise,解析成JSON,具体请看下面返回的数据
})
.then(function (res) {
console.log(res); //获取json数据
})
.catch(function (error) {
console.log(error); //请求错误时返回
});
};
修改数据 注意全局更新(put)和局部更新(PATCH)
// 修改数据
const modifyData = () => {
fetch("http://localhost:8001/comments/61", {
// method:'PUT', // 注意这里,这里会全部更新,如果有些参数没写,则默认就会去掉,需要特别注意
method: "PATCH", // 注意这里,这里会局部更新
headers: {
"Content-Type": "application/json",
},
body: window.JSON.stringify({ body: "修改后 some comment" }),
})
.then((res) => {
return res.json(); //返回一个Promise,解析成JSON,具体请看下面返回的数据
})
.then(function (res) {
console.log(res); //获取json数据
})
.catch(function (error) {
console.log(error); //请求错误时返回
});
};
删除数据
// 删除数据
const deleteData = () => {
fetch("http://localhost:8001/comments/61", {
method: "DELETE",
})
.then((res) => {
return res.json(); //返回一个Promise,解析成JSON,具体请看下面返回的数据
})
.then(function (res) {
console.log(res); //获取json数据
})
.catch(function (error) {
console.log(error); //请求错误时返回
});
};
完整代码
import React from "react";
import { Button, Space } from "antd";
export default function HomeIndex() {
// 获取数据
const loadData = () => {
fetch("http://localhost:8001/comments", {
method: "GET",
})
.then((res) => {
return res.json(); //返回一个Promise,解析成JSON,具体请看下面返回的数据
})
.then(function (res) {
console.log(res); //获取json数据
})
.catch(function (error) {
console.log(error); //请求错误时返回
});
};
// 新增数据
const addData = () => {
fetch("http://localhost:8001/comments", {
method: "POST",
headers: {
"Content-Type": "application/json",
},
body: window.JSON.stringify({ id: 61, body: "some comment", postId: 1 }),
})
.then((res) => {
return res.json(); //返回一个Promise,解析成JSON,具体请看下面返回的数据
})
.then(function (res) {
console.log(res); //获取json数据
})
.catch(function (error) {
console.log(error); //请求错误时返回
});
};
// 修改数据
const modifyData = () => {
fetch("http://localhost:8001/comments/61", {
// method:'PUT', // 注意这里,这里会全部更新,如果有些参数没写,则默认就会去掉,需要特别注意
method: "PATCH", // 注意这里,这里会局部更新
headers: {
"Content-Type": "application/json",
},
body: window.JSON.stringify({ body: "修改后 some comment" }),
})
.then((res) => {
return res.json(); //返回一个Promise,解析成JSON,具体请看下面返回的数据
})
.then(function (res) {
console.log(res); //获取json数据
})
.catch(function (error) {
console.log(error); //请求错误时返回
});
};
// 删除数据
const deleteData = () => {
fetch("http://localhost:8001/comments/61", {
method: "DELETE",
})
.then((res) => {
return res.json(); //返回一个Promise,解析成JSON,具体请看下面返回的数据
})
.then(function (res) {
console.log(res); //获取json数据
})
.catch(function (error) {
console.log(error); //请求错误时返回
});
};
return (
<div>
<Space>
<Button
type="primary"
onClick={() => {
loadData();
}}
>
加载
</Button>
<Button
type="primary"
onClick={() => {
addData();
}}
>
增加
</Button>
<Button
type="primary"
onClick={() => {
modifyData();
}}
>
修改
</Button>
<Button
type="primary"
onClick={() => {
deleteData();
}}
>
删除
</Button>
</Space>
</div>
);
}
其他 API 使用
Based on the previous db.json
file, here are all the default routes. You can also add other routes using --routes
.
Plural routes
GET /posts
GET /posts/1
POST /posts
PUT /posts/1
PATCH /posts/1
DELETE /posts/1
Singular routes
GET /profile
POST /profile
PUT /profile
PATCH /profile
Filter
使用.
操作 对象属性值,比如访问更深层次的属性
GET /posts?title=json-server&author=typicode
GET /posts?id=1&id=2
GET /comments?author.name=typicode
Paginate
使用 _page
和可选的 _limit
来对返回数据定制(不设置默认返回10条
)。
在返回的Response Headers
中,有一个属性Link
,里面会有first
, prev
, next
and last links
。
GET /posts?_page=7
GET /posts?_page=7&_limit=20
10 items are returned by default
Sort
使用 _sort
和 _order
(默认是 ascending)
GET /posts?_sort=views&_order=asc
GET /posts/1/comments?_sort=votes&_order=asc
对于多字段的排序, 可以这样
GET /posts?_sort=user,views&_order=desc,asc
Slice
使用 _start
和 _end
或者 _limit
(an X-Total-Count
header is included in the response)
GET /posts?_start=20&_end=30
GET /posts/1/comments?_start=20&_end=30
GET /posts/1/comments?_start=20&_limit=10
Works exactly as Array.slice (i.e. _start
is inclusive and _end
exclusive)
Operators
使用 _gte
或 _lte
选取一个范围
GET /posts?views_gte=10&views_lte=20
使用 _ne
排除一个值
GET /posts?id_ne=1
使用 _like
进行模糊查找 (支持正则表达式)
GET /posts?title_like=server
Full-text search (全文检索)
使用 q
,在对象全部 value 中遍历查找包含指定值的数据
GET /posts?q=internet
Relationships (关系图谱)
获取包含下级资源的数据, 使用 _embed
GET /posts?_embed=comments
GET /posts/1?_embed=comments
获取包含上级资源的数据, 使用 _expand
GET /comments?_expand=post
GET /comments/1?_expand=post
To get or create nested resources (by default one level, add custom routes for more)
GET /posts/1/comments
POST /posts/1/comments
Database
GET /db
Homepage
Returns default index file or serves ./public
directory
GET /
json-server github 地址 : https://github.com/typicode/json-server
延申出两个问题:
-
json-server 和 mock 有什么区别?
-
json-server 和 mock 是否能共同使用?